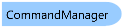
'Declaration
Public Class CommandManager
'Usage
Dim instance As CommandManager
public class CommandManager
Each workbook set is associated with one instance of CommandManager. This association is established when the CommandManager constructor is called.
To create a custom undoable command:
CommandCustom command = new CommandCustom();
workbookView1.ActiveCommandManager.Execute(command);
To override the behavior of an existing command:
/* * Create an instance of CommandSimple and execute it. */ private void buttonExecute_Click(object sender, EventArgs e) { // Acquire a lock on the workbook set. workbookView.GetLock(); try { // Create a simple command. CommandSimple command = new CommandSimple(workbookView); // Execute the command. workbookView.ActiveCommandManager.Execute(command); } finally { // Release the lock on the workbook set. workbookView.ReleaseLock(); } } /* * Undo the last executed command if there is one. */ private void buttonUndo_Click(object sender, EventArgs e) { // Acquire a lock on the workbook set. workbookView.GetLock(); try { // Undo the last undoable command. workbookView.ActiveCommandManager.Undo(); } catch (Exception exc) { MessageBox.Show( this, exc.Message, "Exception", MessageBoxButtons.OK, MessageBoxIcon.Error); } finally { // Release the lock on the workbook set. workbookView.ReleaseLock(); } } /* * Demonstrate creating a simple undoable command which * toggles the display of gridlines. */ private class CommandSimple : SpreadsheetGear.Commands.Command { private WorkbookView _workbookView; private bool _saveDisplayGridlines; public CommandSimple(WorkbookView workbookView) : base(workbookView.ActiveWorkbook) { _workbookView = workbookView; } public override string DisplayText { get { // Text displayed in Undo menu. return "Toggle Display Gridlines"; } } public override CommandUndoSupport UndoSupport { get { // Undo is fully supported. return CommandUndoSupport.Full; } } protected override bool Execute() { // Verify that a lock has already been acquired. System.Diagnostics.Debug.Assert(_workbookView.ActiveWorkbookSet.HasLock); // Execute the command - we won't save state since we're // just toggling a boolean value. IWorksheetWindowInfo windowInfo = _workbookView.ActiveWorksheetWindowInfo; _saveDisplayGridlines = windowInfo.DisplayGridlines; windowInfo.DisplayGridlines = !_saveDisplayGridlines; return true; } protected override bool Undo() { // Undo the command. _workbookView.ActiveWorksheetWindowInfo.DisplayGridlines = _saveDisplayGridlines; return true; } }
System.Object
SpreadsheetGear.Commands.CommandManager
Target Platforms: Windows 7, Windows Vista SP1 or later, Windows XP SP3, Windows Server 2008 (Server Core not supported), Windows Server 2008 R2 (Server Core supported with SP1 or later), Windows Server 2003 SP2